SANS Holiday Hack Challenge™ 2024 - Elf Connect
Join Angel Candysalt in the 2024 Sans Holiday Hack Challenge! Connect the dots in this thrilling puzzle game, where every move counts. Ready for a mind-bending experience? Let's solve it together!
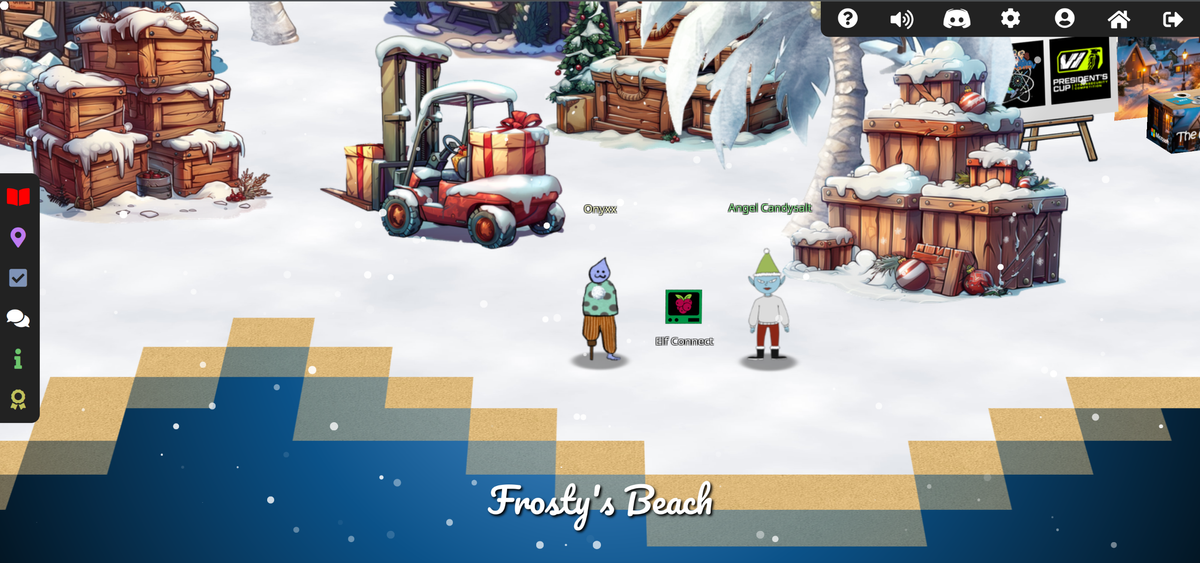
Help Angel Candysalt connect the dots in a game of connections.
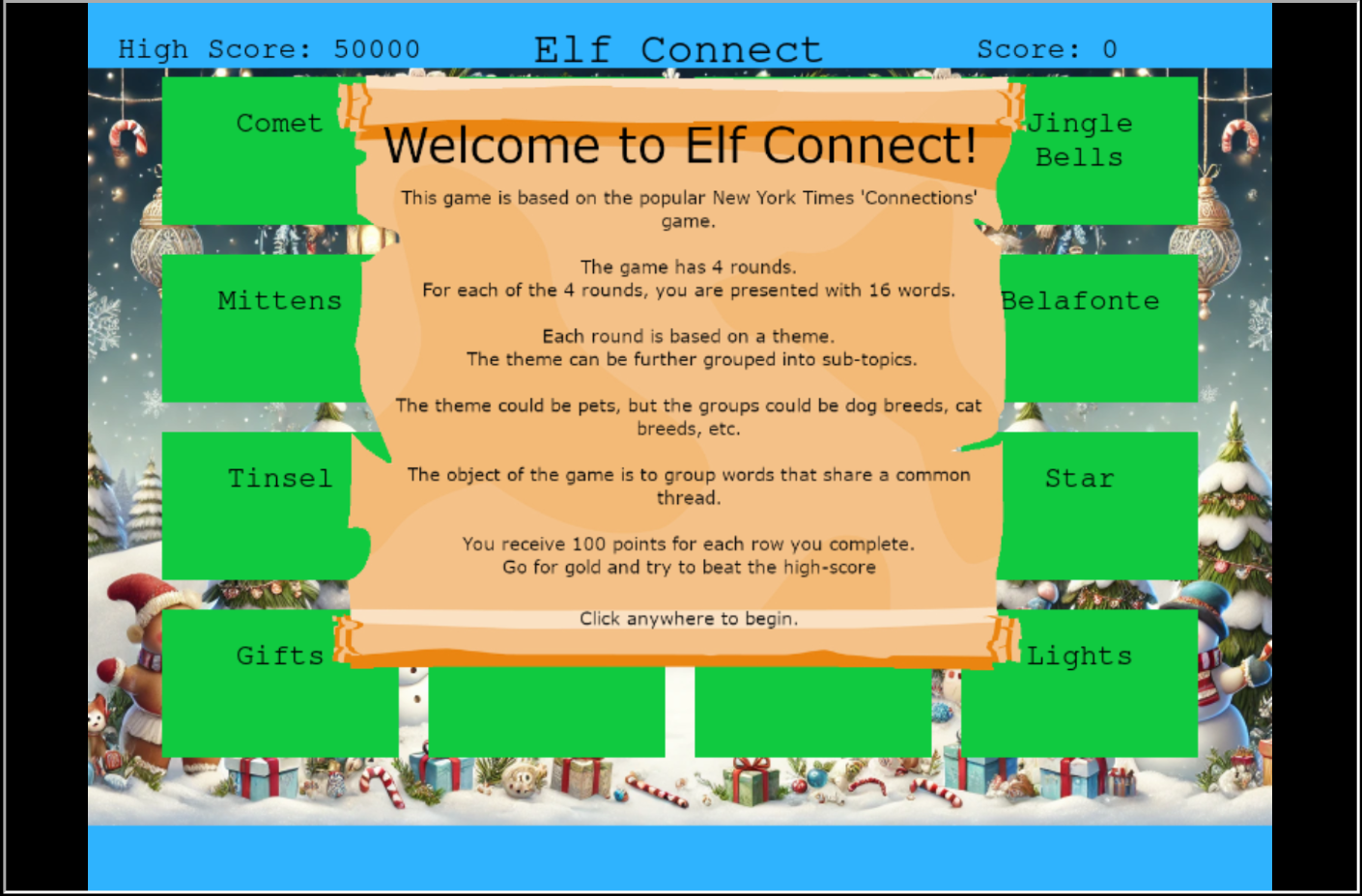
The challenge: uncovering the link between four seemingly related words.
Silver Trophy Challenge
We dive into the console and scan the files (.html
, .js
). One .js
file stands out—it’s a jackpot, the answers are already there!

.js
file!Extracting the contents, we uncover this:
const wordSets = {
1: ["Tinsel", "Sleigh", "Belafonte", "Bag", "Comet", "Garland", "Jingle Bells", "Mittens", "Vixen", "Gifts", "Star", "Crosby", "White Christmas", "Prancer", "Lights", "Blitzen"],
2: ["Nmap", "burp", "Frida", "OWASP Zap", "Metasploit", "netcat", "Cycript", "Nikto", "Cobalt Strike", "wfuzz", "Wireshark", "AppMon", "apktool", "HAVOC", "Nessus", "Empire"],
3: ["AES", "WEP", "Symmetric", "WPA2", "Caesar", "RSA", "Asymmetric", "TKIP", "One-time Pad", "LEAP", "Blowfish", "hash", "hybrid", "Ottendorf", "3DES", "Scytale"],
4: ["IGMP", "TLS", "Ethernet", "SSL", "HTTP", "IPX", "PPP", "IPSec", "FTP", "SSH", "IP", "IEEE 802.11", "ARP", "SMTP", "ICMP", "DNS"]
};
let wordBoxes = [];
let selectedBoxes = [];
let correctSets = [
[0, 5, 10, 14], // Set 1
[1, 3, 7, 9], // Set 2
[2, 6, 11, 12], // Set 3
[4, 8, 13, 15] // Set 4
];
Manually counting could work—like for the first word set: ['Tinsel', 'Garland', 'Star', 'Lights']. But why not save time with a simple script to compute all the correct sets?
# Define word sets
word_sets = {
1: ["Tinsel", "Sleigh", "Belafonte", "Bag", "Comet", "Garland", "Jingle Bells", "Mittens", "Vixen", "Gifts",
"Star", "Crosby", "White Christmas", "Prancer", "Lights", "Blitzen"],
2: ["Nmap", "burp", "Frida", "OWASP Zap", "Metasploit", "netcat", "Cycript", "Nikto", "Cobalt Strike", "wfuzz",
"Wireshark", "AppMon", "apktool", "HAVOC", "Nessus", "Empire"],
3: ["AES", "WEP", "Symmetric", "WPA2", "Caesar", "RSA", "Asymmetric", "TKIP", "One-time Pad", "LEAP", "Blowfish",
"hash", "hybrid", "Ottendorf", "3DES", "Scytale"],
4: ["IGMP", "TLS", "Ethernet", "SSL", "HTTP", "IPX", "PPP", "IPSec", "FTP", "SSH", "IP", "IEEE 802.11", "ARP",
"SMTP", "ICMP", "DNS"]
}
# Define correct sets
correct_sets = [
[0, 5, 10, 14], # Set 1
[1, 3, 7, 9], # Set 2
[2, 6, 11, 12], # Set 3
[4, 8, 13, 15] # Set 4
]
# Map each word set key to its correct set mappings
for key, words in word_sets.items():
print(f"Word Set {key}:")
for correct_set in correct_sets:
mapped_words = [words[i] for i in correct_set]
print(f" Correct Set: {correct_set} -> {mapped_words}")
The Python script spits out
Word Set 1:
Correct Set: [0, 5, 10, 14] -> ['Tinsel', 'Garland', 'Star', 'Lights']
Correct Set: [1, 3, 7, 9] -> ['Sleigh', 'Bag', 'Mittens', 'Gifts']
Correct Set: [2, 6, 11, 12] -> ['Belafonte', 'Jingle Bells', 'Crosby', 'White Christmas']
Correct Set: [4, 8, 13, 15] -> ['Comet', 'Vixen', 'Prancer', 'Blitzen']
Word Set 2:
Correct Set: [0, 5, 10, 14] -> ['Nmap', 'netcat', 'Wireshark', 'Nessus']
Correct Set: [1, 3, 7, 9] -> ['burp', 'OWASP Zap', 'Nikto', 'wfuzz']
Correct Set: [2, 6, 11, 12] -> ['Frida', 'Cycript', 'AppMon', 'apktool']
Correct Set: [4, 8, 13, 15] -> ['Metasploit', 'Cobalt Strike', 'HAVOC', 'Empire']
Word Set 3:
Correct Set: [0, 5, 10, 14] -> ['AES', 'RSA', 'Blowfish', '3DES']
Correct Set: [1, 3, 7, 9] -> ['WEP', 'WPA2', 'TKIP', 'LEAP']
Correct Set: [2, 6, 11, 12] -> ['Symmetric', 'Asymmetric', 'hash', 'hybrid']
Correct Set: [4, 8, 13, 15] -> ['Caesar', 'One-time Pad', 'Ottendorf', 'Scytale']
Word Set 4:
Correct Set: [0, 5, 10, 14] -> ['IGMP', 'IPX', 'IP', 'ICMP']
Correct Set: [1, 3, 7, 9] -> ['TLS', 'SSL', 'IPSec', 'SSH']
Correct Set: [2, 6, 11, 12] -> ['Ethernet', 'PPP', 'IEEE 802.11', 'ARP']
Correct Set: [4, 8, 13, 15] -> ['HTTP', 'FTP', 'SMTP', 'DNS']
And just like that, the challenge is solved!
Gold Trophy Challenge
By digging through the same .js
file, we spot the score
variable. It pulls and sets the score
and roundComplete
values through session storage, while the round
is determined by the URL parameters (https://URL[.]com/?&challenge=termElfConnect&REDACTED).
let score = parseInt(sessionStorage.getItem('score') || '0'); // Initialize score
let scoreText; // Text object for score display
let highScore = 50000;
let highScoreText; // text object for high score
let roundComplete = sessionStorage.getItem('roundComplete');
if (roundComplete == null) {
roundComplete = 0;
}
// let urlParams = new URLSearchParams(window.location.search);
let round = parseInt(urlParams.get('round') ?? 1, 10); // Default to round 1 if no parameter is set
let words = wordSets[round];
You might consider setting the variables in session storage, but there's a simpler approach since:
let urlParams = new URLSearchParams(window.location.search);
const roundCheck = urlParams.get('round');
if (!roundCheck) { // If 'round' is absent or has no value
sessionStorage.clear();
}
Open the Browser > Console > Context (in FireFox, it shows Top
), then switch the context to Christmas Word Connect
.

Now, enter this into the console:
score=90000
round=4
Then, pick one matching set to update the score, I picked ['Sleigh', 'Bag', 'Mittens', 'Gifts']
:
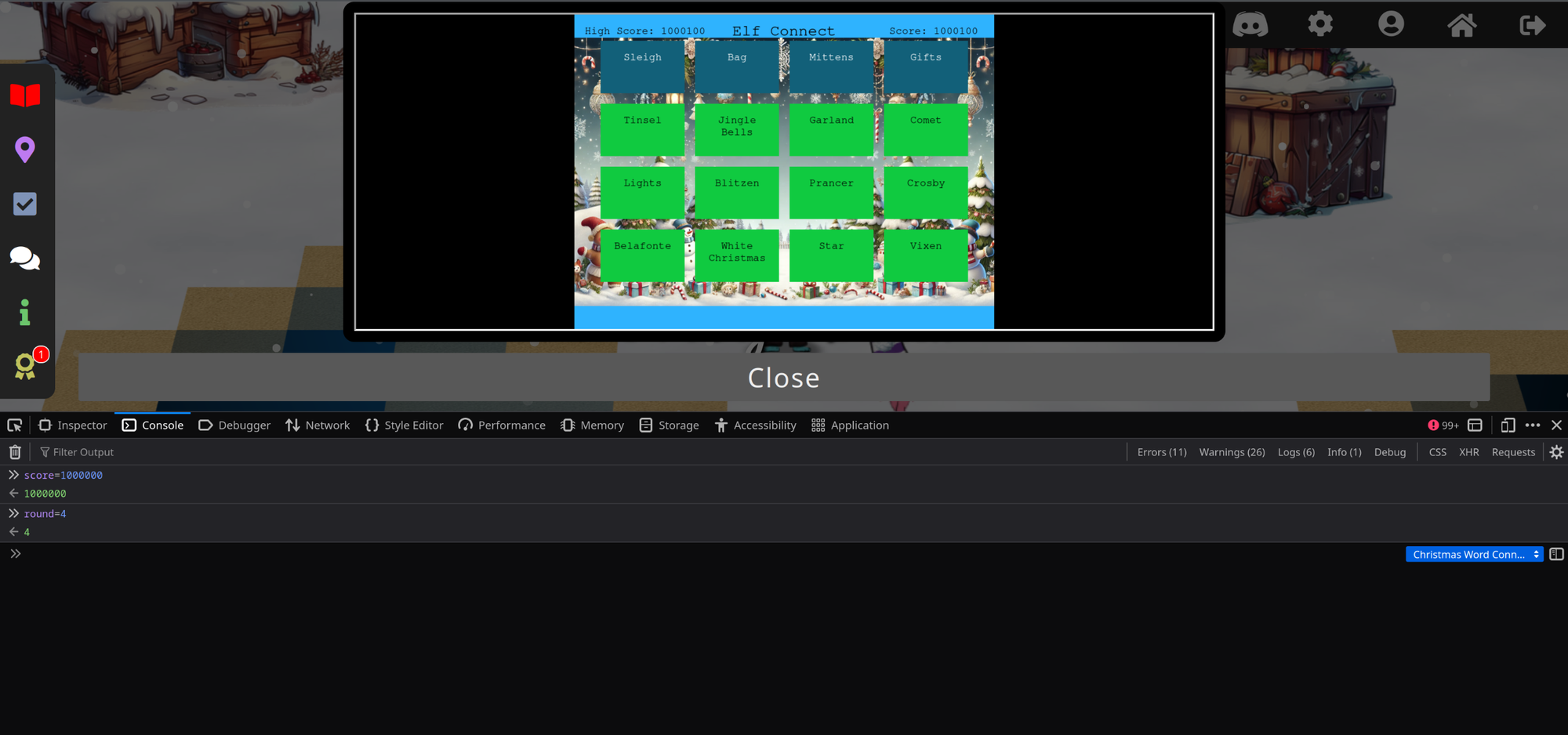
Onto the next challenge!
Comments ()